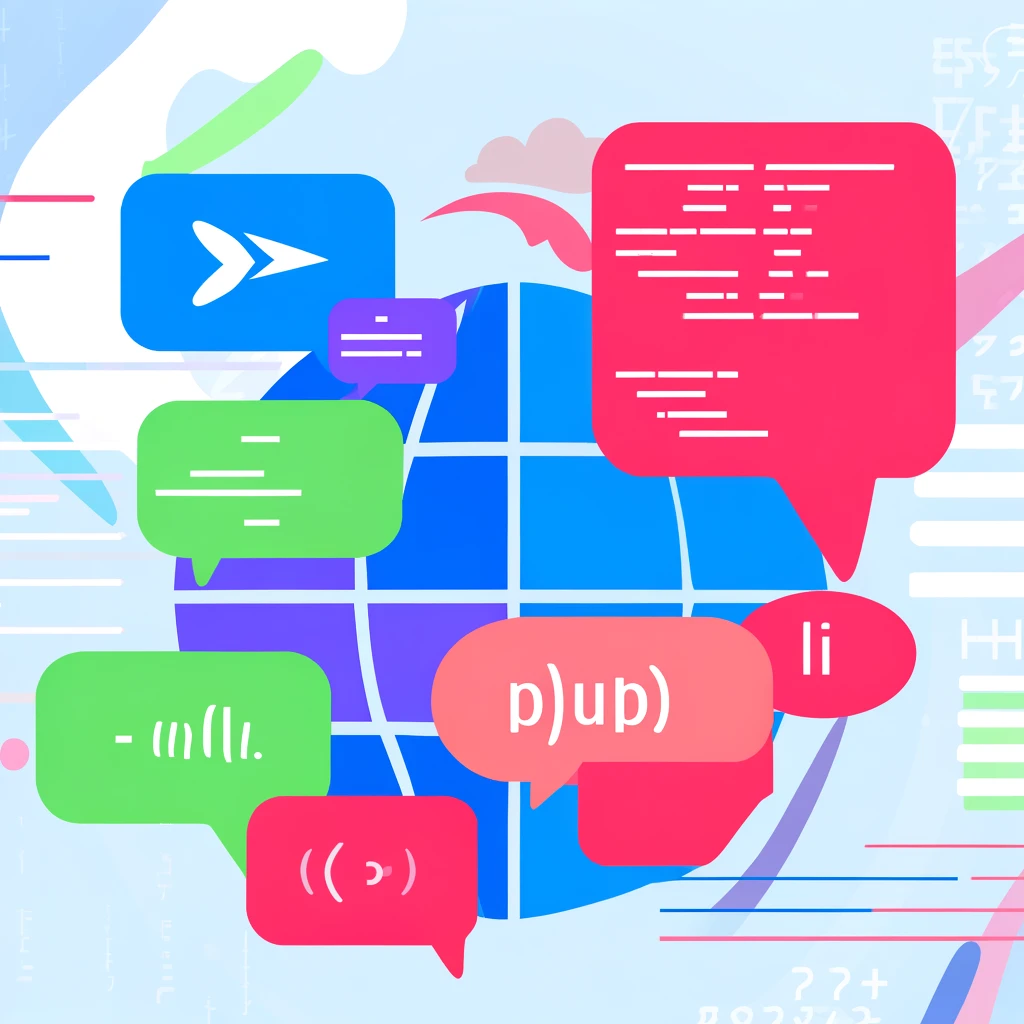
Rails, Internationalization & Pluralization
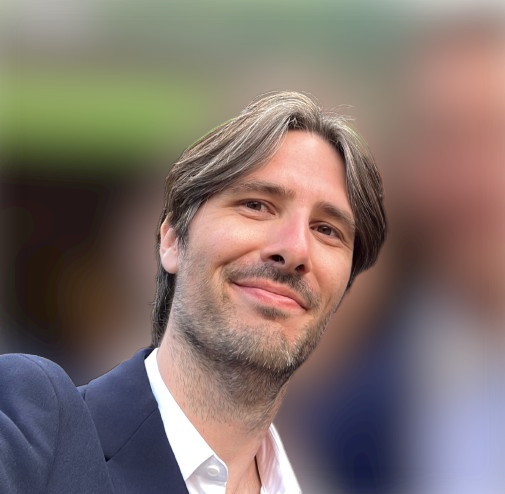
Bleep Bloop! You have 0 credit(s) left!
.
Well, thank you Mister or Miss Web Page from the 2010’s!
Nowadays, we’re closer to the singularity as modern application will simply state You have no credits left
.
Pluralization is a common usecase for modern user interface. As pluralization works differently for some languages, it’s normal to be part of Internationalization (I18n).
I18n is such a common use case that libraries for it are included in all modern frameworks toolbox. Rails also provide a pluralization tool which is crucial to its core. Both deal with language grammar and pluralization but their API is different. So which one to use?
We gonna see how both work and how to make the best use of them.
Rails Conventions
Ruby-on-Rails is optimized for developer happiness. Using the scaffold feature, we can create a phone book in 10 seconds because behind the scene, Rails follows several conventions to make sure everything is wired together. Let’s quickly see what Rails scaffold feature does.
> rails generate scaffold person name:string phone:string
invoke active_record
create db/migrate/20240416030324_create_people.rb
create app/models/person.rb
invoke resource_route
route resources :people
invoke scaffold_controller
create app/controllers/people_controller.rb
invoke erb
create app/views/people
create app/views/people/index.html.erb
create app/views/people/edit.html.erb
create app/views/people/show.html.erb
create app/views/people/new.html.erb
create app/views/people/_form.html.erb
create app/views/people/_person.html.erb
In this example we see that Rails follow the MVC pattern by creating a Person
model which is fetch and served by a People
controller.
class PeopleController < ApplicationController
before_action :set_person, only: %i[ show edit update destroy ]
# GET /people
def index
@people = Person.all
end
# GET /people/1
def show
end
# GET /people/new
def new
@person = Person.new
end
# GET /people/1/edit
def edit
end
# POST /people
def create
@person = Person.new(person_params)
if @person.save
redirect_to @person, notice: "Person was successfully created."
else
render :new, status: :unprocessable_entity
end
end
# PATCH/PUT /people/1
def update
if @person.update(person_params)
redirect_to @person, notice: "Person was successfully updated.", status: :see_other
else
render :edit, status: :unprocessable_entity
end
end
# DELETE /people/1
def destroy
@person.destroy!
redirect_to people_url, notice: "Person was successfully destroyed.", status: :see_other
end
private
# Use callbacks to share common setup or constraints between actions.
def set_person
@person = Person.find(params[:id])
end
# Only allow a list of trusted parameters through.
def person_params
params.require(:person).permit(:name, :phone)
end
end
These controller endpoints are accessible through Restful routes, using the plural form for resources. As shown, Rails automatically pluralizes Person
to People
.
If we started the webserver, we would see that we have a functional web application.
So, how does Rails manage to create a model and integrate everything with the controller and routes? It starts by pluralizing the model name.
Inflection
Rails Inflector API is the library at play here. This module contains a set of methods to manipulate strings and classes. From the module, we can pluralize, singularize, camelize, underscore, humanize, titleize, classify, dasherize, demodulize, tableize, and constantize strings.
That’s a lot of methods! Let’s see how it works.
> CreditCard.name
=> "CreditCard"
> CreditCard.name.pluralize
=> "CreditCards"
> CreditCard.name.underscore
=> "credit_card"
# Table name in db is the snake_case pluralized version of the class name
> CreditCard.name.tableize
=> "credit_cards"
> CreditCard.name.foreign_key
=> "credit_card_id"
# From the controller, we can access the model class
# Obviously, don't do that at home
> MyModule::PeopleController.name.demodulize.underscore.gsub('_controller', '').singularize.titleize.constantize
=> Person
It’s the pluralize
method that we are interested in here. English being the main language used for naming things, the Inflection Module adds an s
to words even if they don’t exist.
'contact'.pluralize # -> 'contacts'
'shoe'.pluralize # -> 'shoes'
'glurbglurb'.pluralize # -> 'glurbglurbs'
But what about irregulars words? Well, as we saw before, Rails is taking care of that.
'fish'.pluralize # -> 'fish'
'person'.pluralize # -> 'people'
Neat! Guess what, it works the other way too! Did you know that last one?
'glurbglurbs'.singularize # -> 'glurbglurb'
'data'.singularize # -> 'datum'
Rails provide a DSL to add our own inflections and provide in the config/initializers/inflections.rb
file with concise doc and examples.
# Be sure to restart your server when you modify this file.
# Add new inflection rules using the following format. Inflections
# are locale specific, and you may define rules for as many different
# locales as you wish. All of these examples are active by default:
# ActiveSupport::Inflector.inflections(:en) do |inflect|
# inflect.plural /^(ox)$/i, "\\1en"
# inflect.singular /^(ox)en/i, "\\1"
# inflect.irregular "person", "people"
# inflect.uncountable %w( fish sheep )
# end
# These inflection rules are supported but not enabled by default:
# ActiveSupport::Inflector.inflections(:en) do |inflect|
# inflect.acronym "RESTful"
# end
So it’s local specific as well. Great! So maybe we don’t need I18n after all?
Rails I18n
I18n is much more than pluralization obviously. The former allows translation for sentences or paragraphs, date and time formating based on local standards and also manage pluralizations. Rails I18n is a key-value store that can be accessed through the I18n
module.
We just have to set the locale gloabaly and call the key we want to translate with the t
method. We can as well localize dates with the l
.
I18n.t('hello') # -> 'Hello world'
I18n.t('hello', locale: :fr) # -> 'Bonjour le monde'
I18n.l(Time.now, format: :long) # -> 'March 19, 2024 12:00'
I18n.locale = :fr
I18n.t('hello') # -> 'Bonjour le monde'
I18n.l(Time.now, format: :long) # -> '19 mars 2024 12:00'
Translations are stored in the config/locales
folder with a file per locale. The key-value store is a hash with the key being the key and the value being the translation.
en:
hello: 'Hello world'
date:
formats:
long: "%B %d, %Y %H:%M"
fr:
hello: 'Bonjour le monde'
date:
formats:
long: "%d %B %Y %H:%M"
So how does I18n manage pluralization? Well, it’s quite simple. We just have to add a count
key to the translation and the value will be a hash with the plural form as key and the translation as value. Depending of the language, plural rules can be different. To categorize them, the CLDR (Common Locale Data Repository) provides a list of plural rules.
en:
credit:
zero: 'You have no credits left'
one: 'You have 1 credit left'
other: 'You have %{count} credits left'
ru:
credit:
zero: 'У вас нет кредитов'
one: 'У вас 1 кредит'
few: 'У вас %{count} кредита'
many: 'У вас %{count} кредитов'
other: 'У вас %{count} кредита'
And we can call it with the t
method with the count
key.
I18n.t('credit', count: 0) # -> 'You have no credits left'
I18n.t('credit', count: 1) # -> 'You have 1 credit left'
I18n.t('credit', count: 5) # -> 'You have 5 credits left'
I18n.locale = :ru
I18n.t('credit', count: 0) # -> 'У вас нет кредитов'
I18n.t('credit', count: 1) # -> 'У вас 1 кредит'
I18n.t('credit', count: 5) # -> 'У вас 5 кредитов'
That’s not all, we can also translate models and attributes with the activerecord
scope.
fr:
activerecord:
models:
person: 'Personne'
attributes:
person:
name: 'Nom'
And access the translation from the model class.
Person.model_name.human # -> 'Personne'
Person.human_attribute_name(:name) # -> 'Nom'
And now, let’s pluralize our model.
en:
activerecord:
models:
credit:
one: 'Credit'
other: 'Credits'
person:
one: 'Person'
other: 'People'
Let’s see if it works.
Credit.model_name.human # -> 'Credit'
Credit.model_name.human(count: 2) # -> 'Credits'
Person.model_name.human # -> 'Person'
Person.model_name.human(count: :plural) # -> 'People'
It works! But something is off. Why do we have to use the count
key? At least, we can write :plural
(or anything which is not 1
, other
works as a fallback) instead of 2
.
Also we have to specify all the plural forms in the translation file even for the regulars plurals.
In most case, the intent is clearer by using the pluralize
method from the Inflection
module.
en:
activerecord:
models:
person: 'Person'
fr:
activerecord:
models:
person: 'Personne'
Person.model_name.human # -> 'Person'
Person.model_name.human.pluralize # -> 'People'
I18n.locale = :fr
Person.model_name.human # -> 'Personne'
Person.model_name.human.pluralize # -> 'Personnes'
It’s more readable and it’s consistent with the Rails conventions and it works, right? WRONG!
The Ugly Part
French and English share a lot of similarities like adding an s
to pluralize a word in most cases. But same as English, French has irregulars plurals. So let’s try to pluralize Horse
in French.
en:
activerecord:
models:
horse: 'Horse'
fr:
activerecord:
models:
horse:
one: 'Cheval'
other: 'Chevaux'
Horse.model_name.human # -> 'Horse'
Horse.model_name.human.pluralize # -> 'Horses'
I18n.locale = :fr
Horse.model_name.human # -> 'Cheval'
Horse.model_name.human.pluralize # -> 'Chevals'
Nope! It’s not working. The pluralize
method is treating cheval
and glurbglurb
the same way.
So what’s the solution? Well, we can use the I18n
module with the count
key, or we can use the convenient DSL provided by the Inflection
module.
ActiveSupport::Inflector.inflections(:fr) do |inflect|
inflect.irregular "cheval", "chevaux"
end
Horse.model_name.human # -> 'Horse'
Horse.model_name.human.pluralize # -> 'Horses'
I18n.locale = :fr
Horse.model_name.human # -> 'Cheval'
Horse.model_name.human.pluralize # -> 'Chevals'
Still not working! The Inflection
module is not taking into account the locale. When we check the documentation, we read “If passed an optional locale parameter, the word will be pluralized using rules defined for that language. By default, this parameter is set to :en.”.
Bummer, so me have to specify the locale each time we pluralize a word.
Horse.model_name.human # -> 'Horse'
Horse.model_name.human.pluralize(I18n.locale) # -> 'Horses'
I18n.locale = :fr
Horse.model_name.human # -> 'Cheval'
Horse.model_name.human.pluralize(I18n.locale) # -> 'Chevaux'
It works now, but at what cost? We have to specify the locale each time we pluralize a word. It’s confusing and not DRY.
Conclusion
Rails inflections are used for the framework internal logic. That’s mainly the reason why it works in English by default. So even though it’s more readable and concise, it’s better to leave it for the framework and use the Rails I18n for pluralization. Rails I18n, on the other hand, is more flexible and can be used for pluralization in any language.
DO
Person.model_name.human(count: :plural)
DON’T
Person.model_name.human.pluralize